Building dynamic activities in Workflow foundation 4 – Part 2
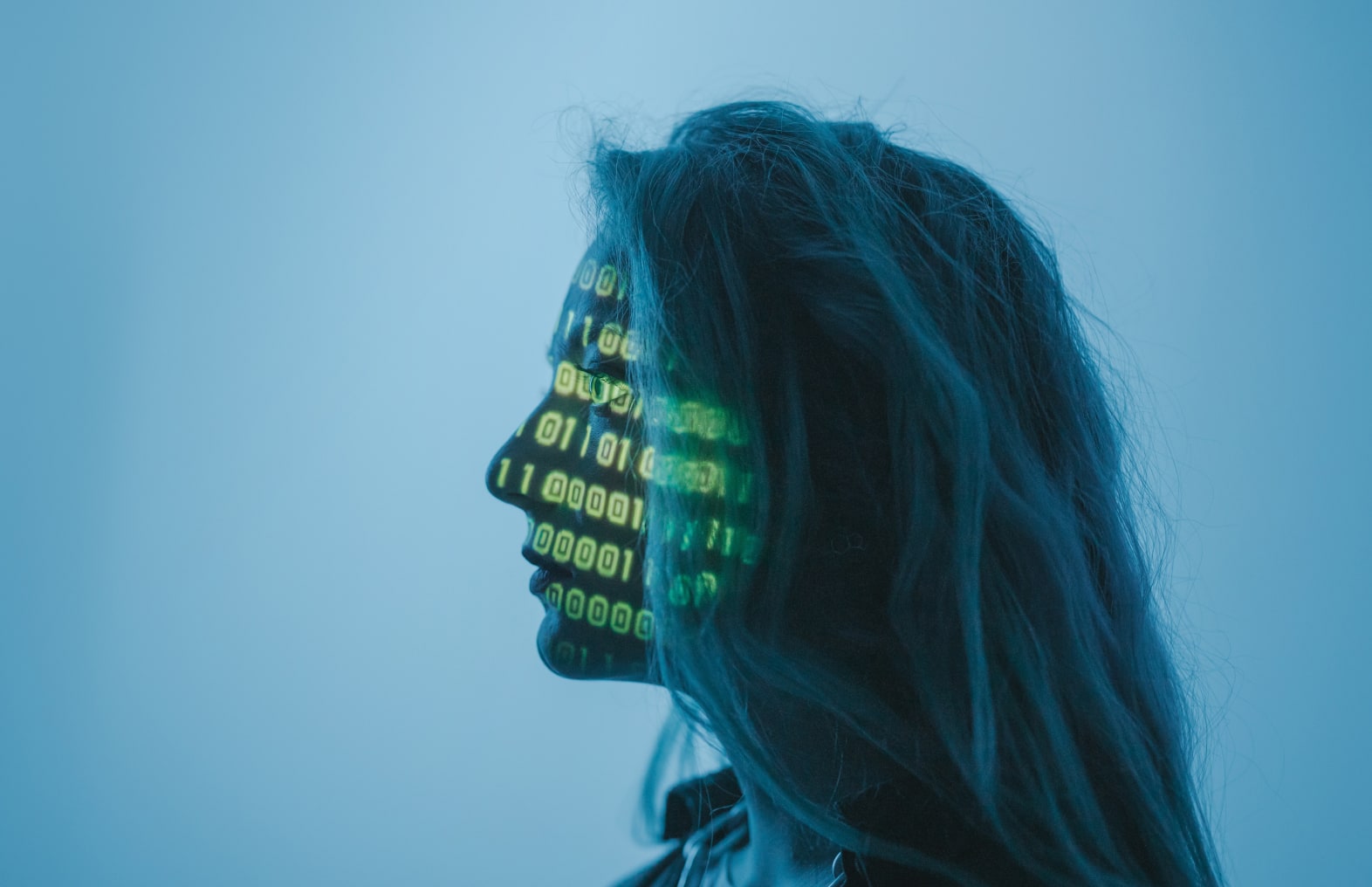
In the previous part I talked about how to develop a dynamic activity by changing just the body of the activity. In this post I will show you how to develop a dynamic activity using the DynamicActivity base class. For those who missed part 1, you can find it here: https://www.infosupport.com/blogs/willemm/archive/2010/09/27/building-dynamic-activities-in-workflow-foundation-4.aspx
Building a dynamic activity using the DynamicActivity class
Creating an activity based on the DynamicActivity class is a little different from the other scenarios. You can’t actually derive from DynamicActivity, because it’s a sealed class. Instead you need to construct the dynamic activity from a factory class. This makes the DynamicActivity one of the most interesting forms of dynamic activities.
To understand how the process of creating a dynamic activity works, you first need to know a little something about the workflow designer in Visual Studio 2010. There are two types of things you can drop onto the workflow designer service. First there’s activities, when you drop those onto the designer, they will get integrated into the workflow you’re designing. There’s nothing special to this scenario as you might expect. The second thing you can drop onto the designer is an instance of IActivityTemplateFactory. This is a special kind of object, that doesn’t represent an activity, but instead produces an activity that the designer will integrate into the workflow you’re designing.
To build an activity that derives from DynamicActivity you need to create a class that implements the IActivityTemplateFactory interface. The basic implementation of such an activity factory looks like the sample below:
1: public class DynamicSendAndReceiveReply : IActivityTemplateFactory
2: {
3: /// <summary>
4: /// Creates the dynamic receive and send activity
5: /// </summary>
6: /// <param name="target">The target.</param>
7: /// <returns></returns>
8: public System.Activities.Activity Create(System.Windows.DependencyObject target)
9: {
10: SelectOperationDialog dlg = new SelectOperationDialog();
11:
12: if (dlg.ShowDialog() == true)
13: {
14: DynamicActivity dynamicSendActivity = new DynamicActivity
15: {
16: Implementation = () => CreateSendActivity()
17: };
18:
19: DynamicActivity dynamicReceiveActivity = new DynamicActivity
20: {
21: Implementation = () => CreateReceiveActivity()
22: };
23:
24: Sequence result = new Sequence
25: {
26: Activities =
27: {
28: dynamicReceiveActivity,
29: dynamicSendActivity
30: }
31: };
32:
33: return result;
34: }
35:
36: return null;
37: }
38:
39: // ... The rest of the factory class
40: }
Within the activity factory you can a wide range of things. For example, you can ask the user to select a WCF service contract and operation and generate the right activity with a specific implementation and properties to invoke the selected method on the WCF service.
Features you will get by using the DynamicActivity class
Creating a DynamicActivity using the method described in the previous section allows two things. First you can define the implementation based on the input provided by the developer who dragged the activity template factory onto the designer. Second you can modify the parameters of the activity based on the input from the developer. In short, this method of building a dynamic activity is very powerful.
Limitations imposed by this design
There’s one down side to this method of building a dynamic activity. Once the template factory produced the activity, it can’t be changed. Unless the developer deletes the activity from the workflow and drags a new instance of the activity template factory from the toolbox onto the designer.
Conclusion
In this part I talked about building a dynamic activity using the DynamicActivity class and the IActivityTemplateFactory class. It offers a powerful mechanism to build dynamic activities, with not a lot of complexity in terms of components needed to provide a good development experience.
In the next part I will show you one last method to build a dynamic activity.