Flash Builder 4: The easiest way to create a combined Flex / Java project
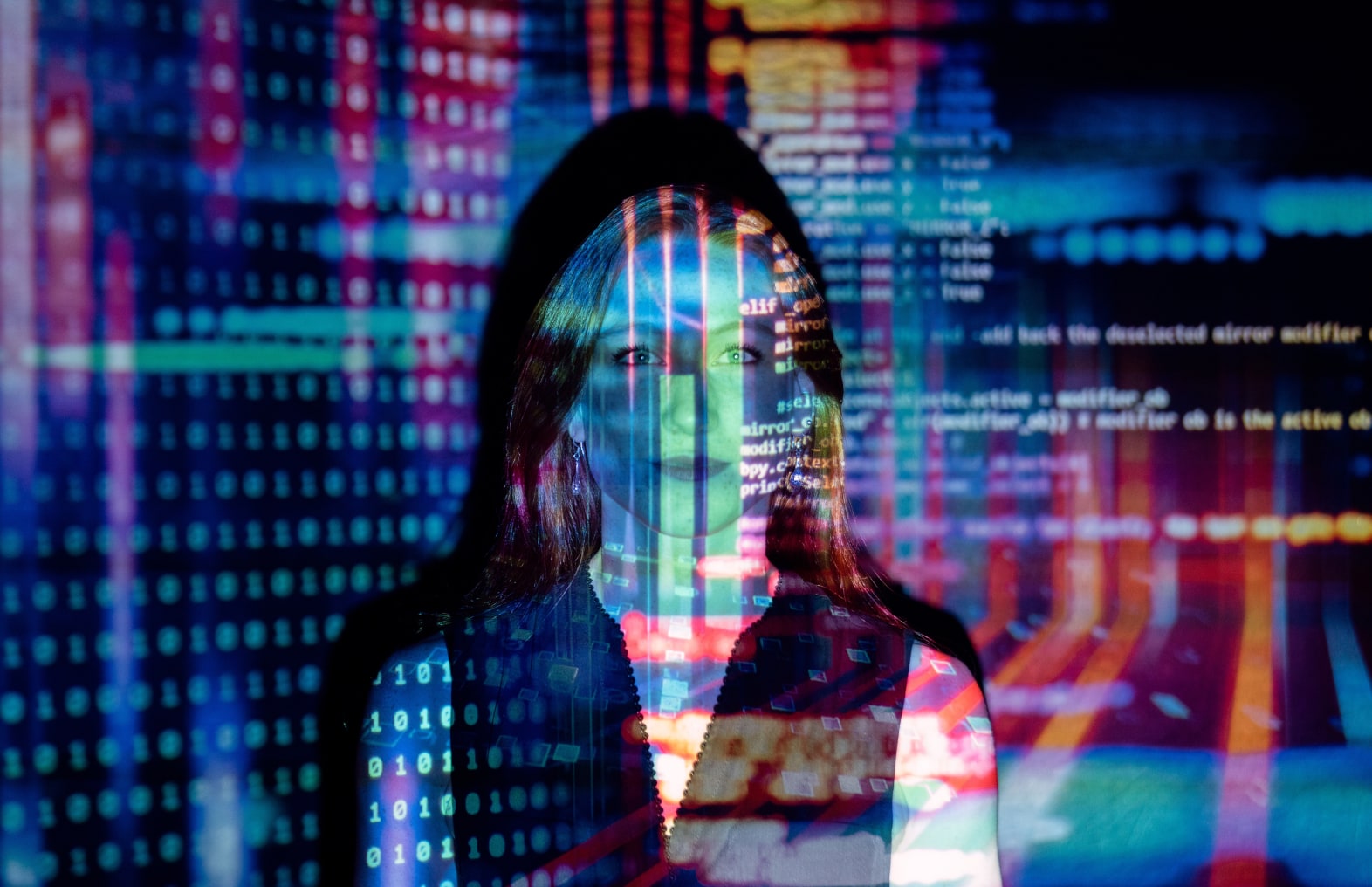
If you have the plugin version of Flash Builder 4 and an Eclipse with the WTP feature installed, you can create a combined Java / Flex project. If you do this the default way, there is a nasty bug which prevents your Flex application from communicating with your Java backend. In this article I will show how you can work around this problem.
Prerequisites
To follow along with this article you need to have the following installed:
- Eclipse 3.5, with WTP. The easiest way is to get this is to get it here. The link will take you to the Eclipse JEE bundle.
- Flash Builder 4 plugged into the above version of Eclipse. You can get a trial version here. Just install it and point it during installation to the location of Eclipse.
- LCDS or BlazeDS which you can get here or here. The first link is for LCDS 3.1 and the second link is for BlazeDS 4. Both require you to sign in with your Adobe id, after which you can download the goods. Note that if you don’t provide a license key during the LCDS installation, you get the developer license which you can use to develop your application, without any feature restrictions. If you don’t have an application server, choose to install Tomcat, which is bundled with both BlazeDS and Eclipse.
Setting up Eclipse
Let’s start up Eclipse, double click on the executable in the location in which you installed the Eclipse JEE bundle, not the executable under “c:Program filesAdobe” etc. It’s important to understand that since you installed Flash Builder as a plugin, your JEE bundle Eclipse is used. This is also important when installing other plugins like the fiber modeler plugin. You need to extract plugins to the plugins folder of your original Eclipse version, not the one which get’s installed by the Flash Builder 4 plugin. Yeah, even if you install Flash Builder 4 as a plugin, there is still a new standalone Flash Builder installed onto your harddrive. For Windows it’s installed in “C:Program FilesAdobeAdobe Flash Builder 4 Plug-ineclipse-host-distro”. For the last time, don’t use this Eclipse, but the Eclipse your Flash Builder 4 plugin has plugged into.
After firing up your original Eclipse, you need to configure a Server first. For this article I’m assuming that you use the bundled Tomcat server. If you use another app server, the steps will be the same, only the location of the app server will be different.
In Eclipse click on “Window”->”Show view”->”Other”. This brings up the following screen:
Expand the “Server” folder, select “Servers” and click “Ok”. after this, you will have the “Servers” view, which is selected on the image below:
Right click on the white background in the view and choose “New”->”Server”. This will bring up the following screen:
Select “Tomcat v6.0 Server” or the app server you’re using instead. When it’s the first time you configure this, you need to add a server runtime environment by clicking “Add” next to the “Server runtime environment” combobox. Configure it like below:
The Tomcat installation directory may differ. Now “Finish” both dialogs. Your Tomcat Server should appear as below:
Now Eclipse is prepared for creating a combined Flex / Java project!
Creating a combined Flex / Java project
The obvious way to create a new Flex / Java project is by clicking “File”->”New”->”Flex project”, which brings up the following screen:
and select “Create combined Java / Flex project using WTP”. This is also the approach that is advocated the most on the web. If you create a combined project this way, there is a nasty bug which prevents your Flex project from communicating with any remote Java objects. Flash Builder get’s the context root of your project all wrong. It should use the “web.context” macro, instead it uses the “web.content” macro. Because of this, your Flex application gets the wrong url to your remote objects compiled in, which can be quite annoying during testing. I submitted this bug to Adobe before Flash Builder was released, but I guess they didn’t had the time to fix it.
The solution:
Don’t create a combined Flex / Java project. Click on “File”->”Import…” and import either the “BlazeDS.war” file or the “LCDS.war” file, which resides in the installation directory of BlazeDS or LCDS:
Then point to the WAR file:
Point to the correct WAR file, choose a cool name for your project and select the earlier configured server. Then click “Finish”. Your project structure should look like this:
What we have now is a Java only web project, which has all the needed BlazeDS or LCDS files. We only need to add a Flex project to it. Right click on the project and choose “Add / Change project type”->”Add Flex project type”. This brings up the following screen:
Configure the screen as shown above, with either “LiveCycle Data Services ES” selected or “BlazeDS”. Then click “Next”. The following screen will appear:
It is of great importance that the screen above is configured correctly. The root folder should point to the “WebContent” folder of your project (the physical folder on your harddrive under your Eclipse workspace), or if you changed the name,the folder with the “WEB-INF” and “META-INF” folders in it. The root URL typically ends with your project name and the context root is the last part of the root URL, minus the trailing “/”. Press “Validate Configuration”, if your server isn’t running you’ll get a warning. Just click “Finish”. Your project structure should look like this:
Note that there is a problem in the “Problems” view. Just right click it and choose “Recreate HTML Templates”. This will fix the error. Then you can add a new “Java Source Folder” to your project which holds your Java classes:
I usually call it “java_src”. Now let’s test our setup. Right click your new Java source folder and add a new Java class:
Click “Finish”. Implement it like this:
1: package testpackage;
2:
3: public class HelloSayer {
4: public String sayHello() {
5: return "Hello from Java!";
6: }
7: }
Now expand your “WebContent/WEB-INF/flex” folder and open the “remoting-config.xml” file. Configure a destination like this:
1: <?xml version="1.0" encoding="UTF-8"?>
2: <service id="remoting-service"
3: class="flex.messaging.services.RemotingService">
4:
5: <adapters>
6: <adapter-definition id="java-object" class="flex.messaging.services.remoting.adapters.JavaAdapter" default="true"/>
7: </adapters>
8:
9: <default-channels>
10: <channel ref="my-amf"/>
11: </default-channels>
12:
13: <destination id="hello">
14: <properties>
15: <source>testpackage.HelloSayer</source>
16: <scope>application</scope>
17: </properties>
18: </destination>
19:
20:
21: </service>
This will make our Java class accessible from Flex. Implement your “MyFirstFlexJavaApp.mxml” file in your “src” folder like this:
1: <?xml version="1.0" encoding="utf-8"?>
2: <s:Application >"http://ns.adobe.com/mxml/2009"
3: >"library://ns.adobe.com/flex/spark"
4: >"library://ns.adobe.com/flex/mx" minWidth="955" minHeight="600">
5:
6: <fx:Script>
7: <![CDATA[
8: import mx.controls.Alert;
9: import mx.rpc.AsyncToken;
10: import mx.rpc.Responder;
11: import mx.rpc.events.FaultEvent;
12: import mx.rpc.events.ResultEvent;
13: import mx.rpc.remoting.RemoteObject;
14: protected function callJava(event:MouseEvent):void
15: {
16: var javaObject : RemoteObject = new RemoteObject("hello");
17: var token : AsyncToken = javaObject.sayHello();
18: token.addResponder(
19: new Responder(
20: handleResult,
21: handleFault
22: )
23: );
24: }
25:
26: private function handleResult(event : ResultEvent) : void {
27: Alert.show(event.result.toString());
28: }
29: private function handleFault(event : FaultEvent) : void {
30: Alert.show(event.fault.message);
31: }
32: ]]>
33: </fx:Script>
34:
35: <s:Button x="280" y="230" label="Contact Java" click="callJava(event)"/>
36: </s:Application>
Now right click your project and choose “Run as”-> “Run on server”. You only have to do this once, to associate your project with your (Tomcat) app server. If you used BlazeDS, the following screen appears:
Verify in the console that your server has started up correctly. Now, right click your project and choose “Run as Web Application”. Your browser should start and your Flex application should display. Click the button to verify that the remote Java object was called:
Concluding
I know, it are still a couple of steps and it’s still not ideal, but the setup above really is the easiest way to create a working combined Java / Flex project. Just try it a couple of times and you’ll get the hang of it :).