Import a GraphQL API in API Management using Powershell
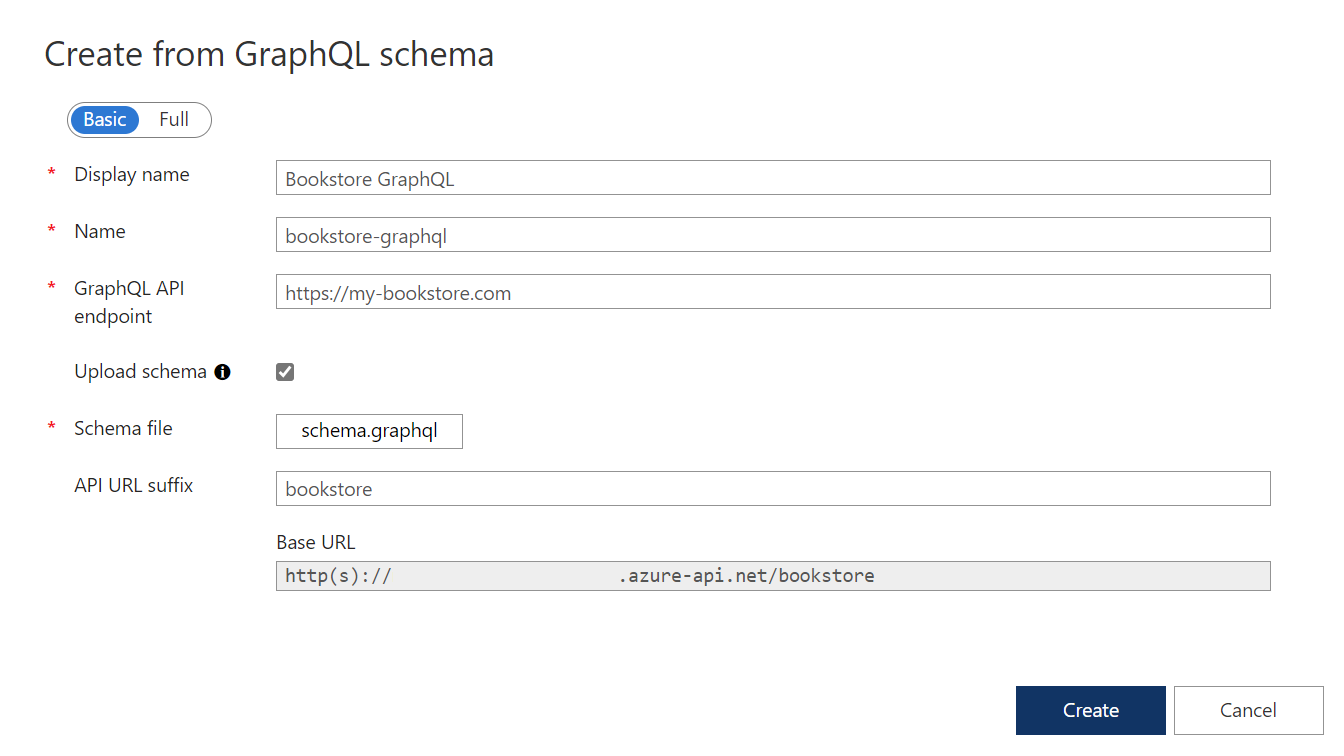
Microsoft has added support for GraphQL passtrough in API Management since the end of 2021. For now (beginning of 2022), it is only possible to import a GraphQL API through the Azure Portal. We have to wait for official Azure CLI support in the future when we want to deploy our APIs programmatically.
So how do the teams at Microsoft do it currently themselves?
In this blogpost, we will take a little look into the inner working of the Azure Portal and create our own deploy script using Powershell.
Taking notes from the Portal
For example, I wanted to create a new Bookstore GraphQL API with a custom schema.
I filled in all the required fields and hit create.
I checked the developer console of the browser to view all network requests and I came across some PUT requests that seemed to have the same data as I previously filled in. One request created a new GraphQL API and another one uploaded my schema.

One of the HTTP requests that created a new GraphQL API.
The Azure CLI and Azure Powershell are wrappers around the Azure REST API. The REST API has more features ahead of its CLI tooling counterparts.
Luckily for us, the CLI tooling has support to directly call the REST API.
In Azure Powershell, we use the Invoke-AzRestMethod cmdlet for this.
I copied the the first HTTP request from the developer console and cleaned it up. After that, I transformed the the request to the following Powershell equivalent.
# Resource specific paramaters $resourceGroupName = '<your-resource-group-name>' $apimServiceName = '<your-apim-name>' # GraphQL API specific paramaters $name = 'bookstore-graphql' $displayName = 'Bookstore GraphQL' $backendUrl = 'https://my-bookstore.com' $path = 'bookstore' $description = 'This is a description of the Bookstore API' $body = @{ id = "/apis/$name" name = $name properties = @{ displayName = $displayName serviceUrl = $backendUrl protocols = @('https') description = $description path = $path type = 'graphql' } } $payload = $body | ConvertTo-Json # Create a new GraphQL API in API Management Invoke-AzRestMethod -ResourceGroupName $resourceGroupName -ResourceType "$apimServiceName/apis" ` -ResourceProviderName 'Microsoft.ApiManagement/service/' -Name $name ` -ApiVersion '2021-04-01-preview' -Method 'PUT' -Payload $payload
After running the this script and checking the Azure Portal, a new API was created in the portal!
The schema was still missing. So I transformed the second HTTP request to Powershell.
# Load a GraphQL SDL schema $schemaPath = "<your-schema-file-path>" $graphqlSchema = Get-Content -Path $schemaPath -Raw $body = @{ properties = @{ contentType = 'application/vnd.ms-azure-apim.graphql.schema' document = @{ value = $graphqlSchema } } } $payload = $body | ConvertTo-Json # Upload a custom GraphQL schema in an existing GraphQL API Invoke-AzRestMethod -ResourceGroupName $resourceGroupName -ResourceType "$apimServiceName/apis/$name/schemas/graphql" ` -ResourceProviderName 'Microsoft.ApiManagement/service/' ` -ApiVersion '2021-04-01-preview' -Method 'PUT' -Payload $payload
Now the schema was also available in the portal!
Until Microsoft has added official support, we can use this solution for now.