Connecting Adobe Flex with Flash Builder 4's Data Centric Development features to a .Net service based backend
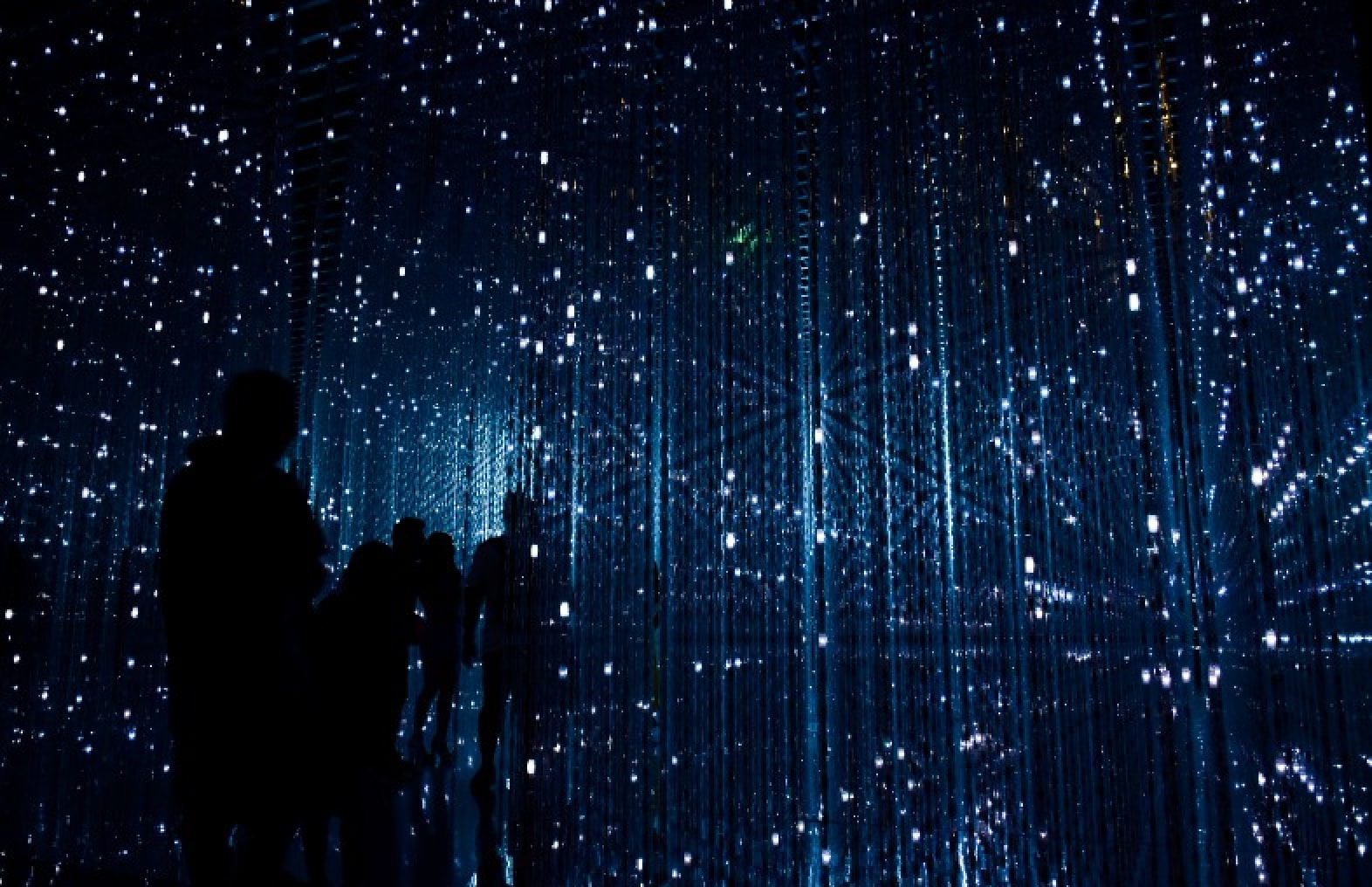
This post is about some of the new Flash Builder 4 Data Centric Development features. I’m going to use these features to integrate a Flex 4 front-end, with a .Net back-end. Let’s start with the .Net back-end.
The .Net Back-end
The .Net back-end will be a WCF Service Application. We’re going to write a webservice which loads employees. Employees are simple objects with two properties, just an Id and a Name will suffice:
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Runtime.Serialization; namespace MyApp { [DataContract] public class Employee { [DataMember] public string Name { get; set; } [DataMember] public int Id { get; set; } } } |
Nothing to fancy about this class…. Next up is the interface “IEmployeeService”:
using System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; namespace MyApp { [ServiceContract] public interface IEmployeeService { [OperationContract] Employee GetEmployee(int id); [OperationContract] Employee[] GetEmployees(); [OperationContract] void UpdateEmployee(Employee emp, Employee original); [OperationContract] int AddEmployee(Employee emp); [OperationContract] void DeleteEmployee(int id); } } |
Notice that we have defined five methods, but four of them are of particular interest:
- GetEmployee, this method will find an Employee by id.
- UpdateEmployee, this method will take two Employees used for updating, first is the updated one, second is the original, used for optimistic concurrency.
- DeleteEmployee, deletes an Employee by id.
- AddEmployee, which adds a newly created Employee to the data store.
Pay attention to the signatures of these four methods, later we will come back to them.
And finally, the implementing class:
using System; using System.Collections.Generic; using System.Linq; using System.Runtime.Serialization; using System.ServiceModel; using System.Text; namespace MyApp { public class EmployeeService : IEmployeeService { private static int _newId = 3; private static List<Employee> _emps =new List<Employee>() { new Employee { Name=“Alex”, Id=1}, new Employee { Name=“Chris”, Id=2}, }; #region IEmployeeService Members public Employee GetEmployee(int id) { return (from e in _emps where e.Id == id select e).Single(); } public Employee[] GetEmployees() { return _emps.ToArray(); } public void UpdateEmployee(Employee emp, Employee original) { Employee old = (from e in _emps where e.Id == emp.Id select e).Single(); if (original.Name != old.Name) { throw new ArgumentException(“Data has been modified”); } old.Name = emp.Name; } public int AddEmployee(Employee emp) { emp.Id = _newId++; _emps.Add(emp); return emp.Id; } public void DeleteEmployee(int id) { _emps.Remove((from e in _emps where e.Id == id select e).Single()); } #endregion } } |
This is a simple class which isn’t thread safe and uses an in memory list to store it’s data for the sake of simplicity. We could have used the Entity Framework, LINQ To SQL, ADO.NET or any other technology of our choosing. You can find the source of the whole WCF Service Application here.
The Flex 4 Application
Now we’re going to build the Flex 4 application, begin with firing up Flash Builder 4 and choose to create a new Flex project. Keep the project settings as follows:
and click finish. Next up, drag a DataGrid onto the design surface and position it nicely. Make sure your EmployeeService is running and click “Data” from the Flash Builder menu bar. The following screen will appear:
Choose WebService and click “Next”. In the following screen, enter the uri to the WSDL of your service and click next again. The final screen will appear:
Click “select all” and click finish. Flash Builder will generate some code for us to call the WebService and will create a strongly typed Employee class. The files that are generated starting with an _ are not for our use, any changes we’ll make to them will be lost when regenerating the code. Instead we can use the empty subclasses generated, which do not start with an _. You should notice that the new “Data / Services” panel (usually below the code / design view) becomes populated. In this panel, click on the getEmployees method and drag the method onto the previously created datagrid in the design view:
After the drag and drop, notice that Flash Builder has generated code to call the webservice in the ”creationComplete” event of the datagrid and has added columns to the datagrid for every property in our Employee class. Start your application and if everything went well, you should see your Employees loaded in your datagrid!
Adding Data Management
But…………. the fun doesn’t stop there! Right click on any operation in the “Data / Services” panel and choose “Enable Data Management”. In the following screen, select the “Id” property and choose “Next”. Make sure the next screen looks like this:
We can only select these methods because of their signatures! Their names are not important, but the Add item for example, should accept one business object and return the newly generated Id. Click finish and Flash Builder will generate some more code. On the surface, it will look like nothing has changed…..
Adding Save functionality
Drag a new button onto the design service and label it “Save”. Put it beneath the datagrid. Make the datagrid editable, but the Id column non-editable. This way users will only be able to change the name. Create a click event handler by right clicking on the button and choose “Generate Click Handler”. Flash Builder will jump to code view. Implement the handler in the following way:
protected function button1_clickHandler(event:MouseEvent):void { employeeService.commit(); } |
Add another button labeled “Cancel”, next to the Save button. Implement the handler for it in the following way:
protected function button2_clickHandler(event:MouseEvent):void { employeeService.revertChanges(); } |
In MXML, bind the “enabled” properties of the two buttons as follows:
enabled=”{employeeService.getDataManager(employeeService.DATA_MANAGER_EMPLOYEE).commitRequired}”
|
This way, users will only be allowed to save or cancel when they’ve made some actual changes! You can find the final Flex application here.
Conclusion
The upcoming release of what is currently known as Flex Builder 3, namely Flash Builder 4, will greatly decrease development time when using Flex in a data oriented manner. In this blog I’ve shown how you can connect with any (web)service based server side technology. The beauty of this all is, Data Management doesn’t only work with webservices, but also with remoting!