Introducing the dialog workspace for Composite WPF
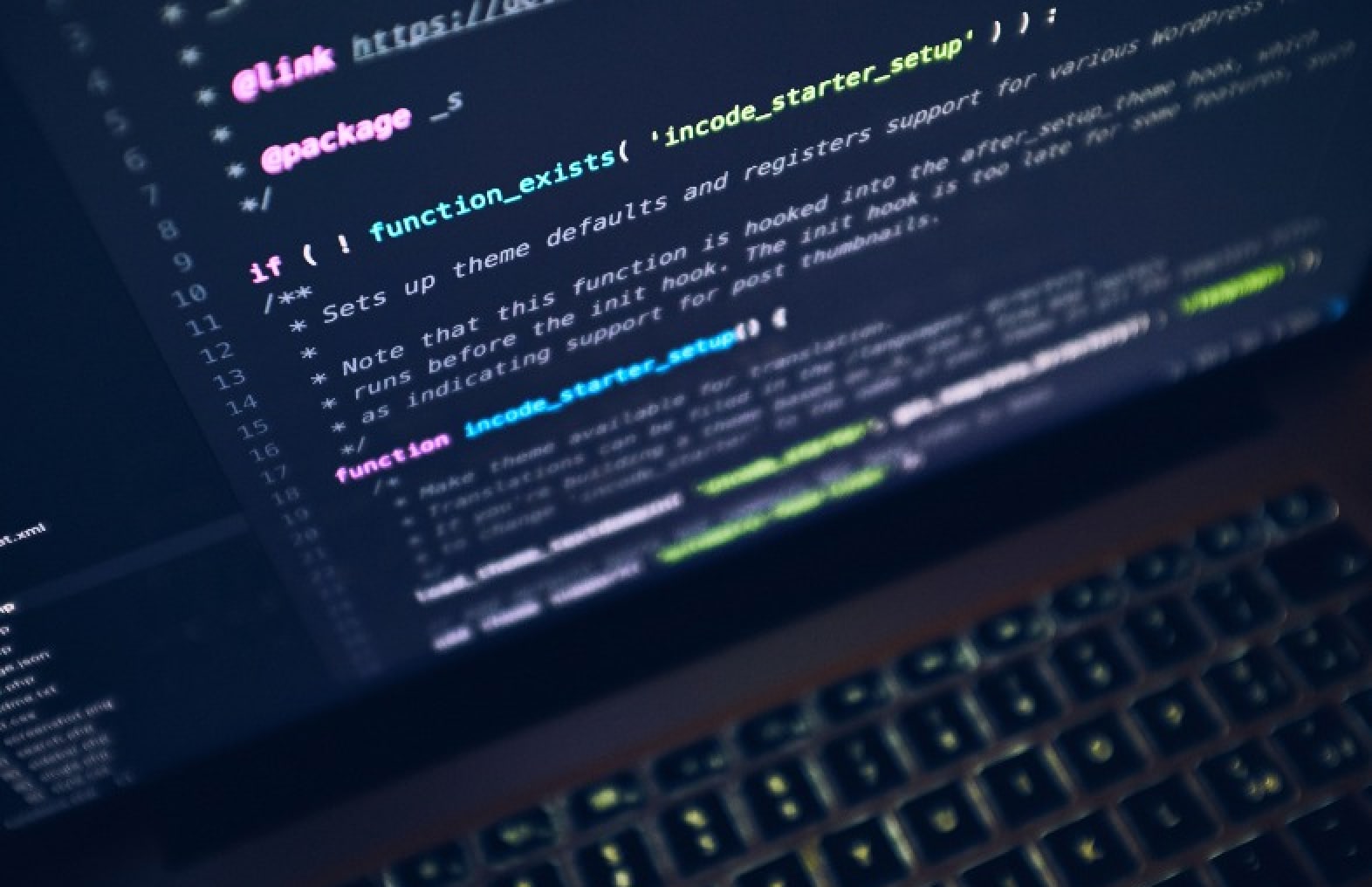
Vacation is the best time to solve some serious puzzles, at least it is for me. The problem I was having with Composite WPF was the fact that I could create composite user interfaces, but there wasn't any support for showing views in dialogs. And because dialogs are widely used I had to come up with a fix for this.In this post I will talk about what the DialogWorkspace class is and how I created it.
What is the dialog workspace?
The dialog Workspace is a class that provides developers with a way to show any view inside a OK/Cancel dialog. This way you can work with a model view presenter pattern and don't have to worry about common infrastructure for the dialog itself. It saves time and you don't have to worry about bugs in the window infrastructure.
Creating a dialog container for a view
The first part of the solution is creating a way to actually show a view inside a dialog. For the first version of the dialog workspace I went with a simple dialog that has a OK and Cancel button on it and some room for the view itself. Pretty simple, but there are a few things that you need to keep in mind when creating the dialog:
- How high?
The view that is going to be displayed in the dialog doesn't have the same size all the time. Every view has its own dimensions and you need to provide a way to resize the dialog to the content. The solution is again simple. By setting the ResizeToContent=WidthAndHeight attribute on the window you can let the window resize when the view is bigger or smaller. - Usability
There's nothing more irritating than not being able to use ENTER to accept the dialog and ESCAPE the cancel the dialog. Most of the time users who use the dialog will want to enter the data quickly and press ENTER to accept the results. This functionality is enabled by setting IsDefault=true for the accept button and IsCancel=True for the cancel button. When the user now presses ENTER or ESCAPE the right button will be "clicked". - The paintjob
WPF does everything in white, at least all the windows are white. But as most developers may know, Windows isn't painted all white. For the most part it has battleship gray in Windows 2003 and a fancier color in Vista and Windows XP. To achieve this you need to add this attribute to the Window element: Background="{DynamicResource {x:Static SystemColors.ControlBrushKey}}"
The workspace
It looks like a really complex class, but it really isn't. The DialogWorkspace class is a static helper class that will configure the dialog and handle the validation logic for you. In this first version it is only possible to provide a view and a delegate to a validation function for the dialog. In the next version I will include more options.
The method that shows the dialog and handles the validation logic looks like this:
/// <summary>
/// Displays a view in the dialog workspace
/// and returns the dialog result after the dialog has been closed
/// </summary>
/// <param name="view">The view to display</param>
/// <param name="validationAction">Validation function for the view</param>
/// <returns>Returns the dialog result of the dialog</returns>
public static bool? ShowView(object view, Func<bool> validationAction)
{
ViewDialog dialog = new ViewDialog();
dialog.View = view as DependencyObject;
dialog.ValidateDialog += new EventHandler<System.ComponentModel.CancelEventArgs>(
(sender, e) =>
{
e.Cancel = false;
if (validationAction != null)
{
// Validate action will return false when validation fails
// invert this result to cancel the close action
e.Cancel = !validationAction();
}
});
return dialog.ShowDialog();
}
Using the Dialog Workspace
Due to the structure of the dialog workspace it is not really that easy to test this component. So instead of creating a unit-test I created a very small WPF application that shows a dialog using the DialogWorkspace class with validation functionality attached. The sample application demonstrates how easy it is to show a view and handle validation logic. The following snippet demonstrates the basics of showing a view as a dialog in the application.
ISampleViewview = _container.Resolve<ISampleView>();
bool? result = DialogWorkspace.ShowView(view,() =>
{
return true;
});
if(result == true)
{
}
Download and more information
As always you can download the latest changeset from http://www.codeplex.com/compositewpfcontrib You can also find more information there on other extensions for Composite WPF.