Using Lambda statement blocks with LINQ May CTP
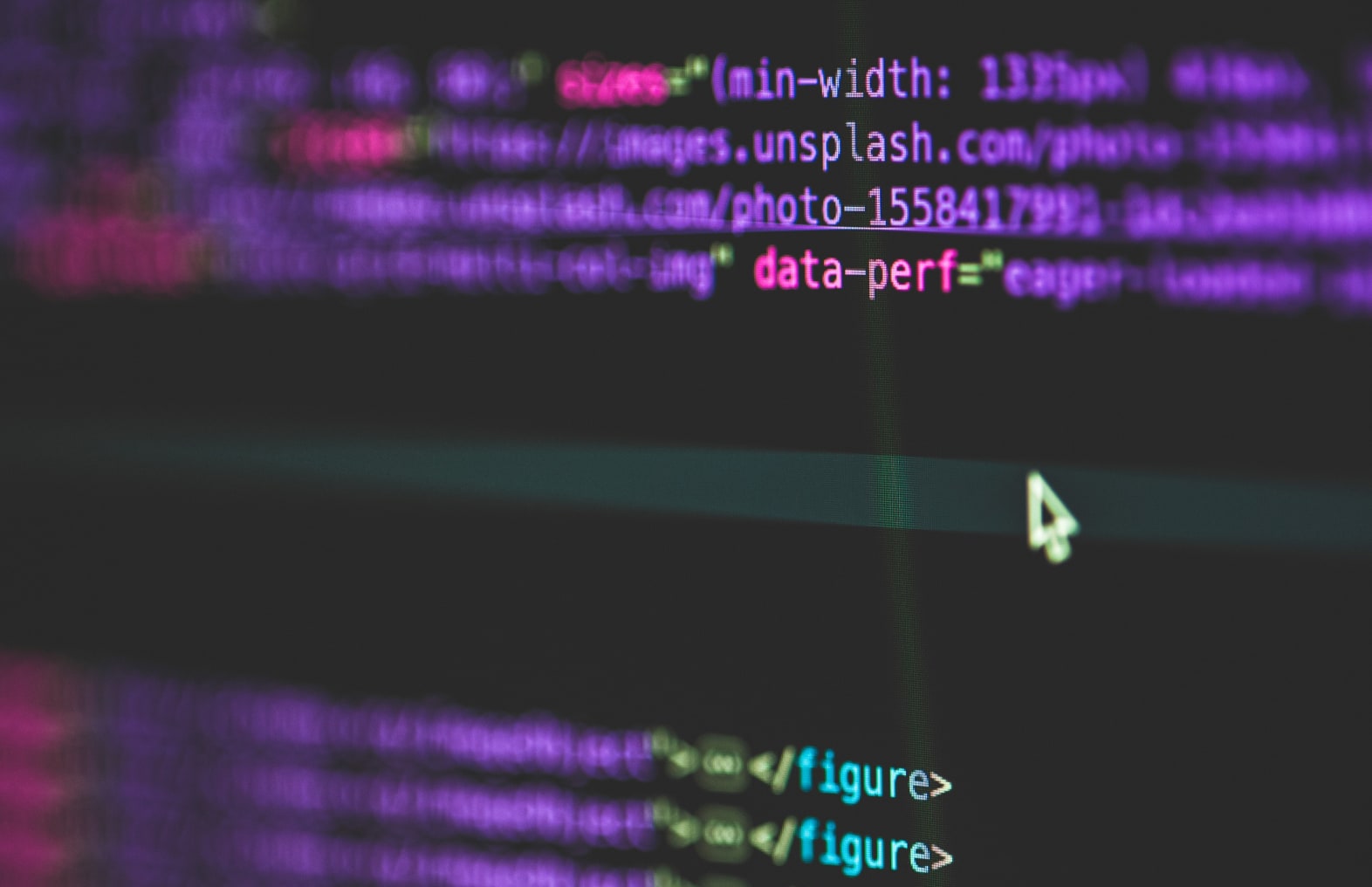
The new LINQ CTP also support lamba statement block, instead of only expressions. Still only expressions are compilable to expression treeds though (hence the name *expression* trees..)
Anyway, this offers the opportunity to do away with anonymous method syntax. See the sample below: no more explicit typing of your parameter, it will be inferred. This certainly makes things more readable
Important to know: The VS compiler does not support this syntax in the IDE yet. I does work however. You should only look at errors that are mentioned in the Output Window
public static class IntegerEx
{
public static IEnumerable<int> UpTo(this int x, int y)
{
for(var i = x; i <= y; i++)
{
yield return i;
}
}
public static void Do(this IEnumerable source, Action action)
{
foreach(T item in source)
{
action(item);
}
}
}
class Program
{
static void Main(string[] args)
{
2.UpTo(6).Do( x =>
{
string message = string.Format("The number is {0}", x);
Console.WriteLine(message);
} );
}
}