Listing mounted ISO's and mounting or unmounting them for VM's in SCVMM
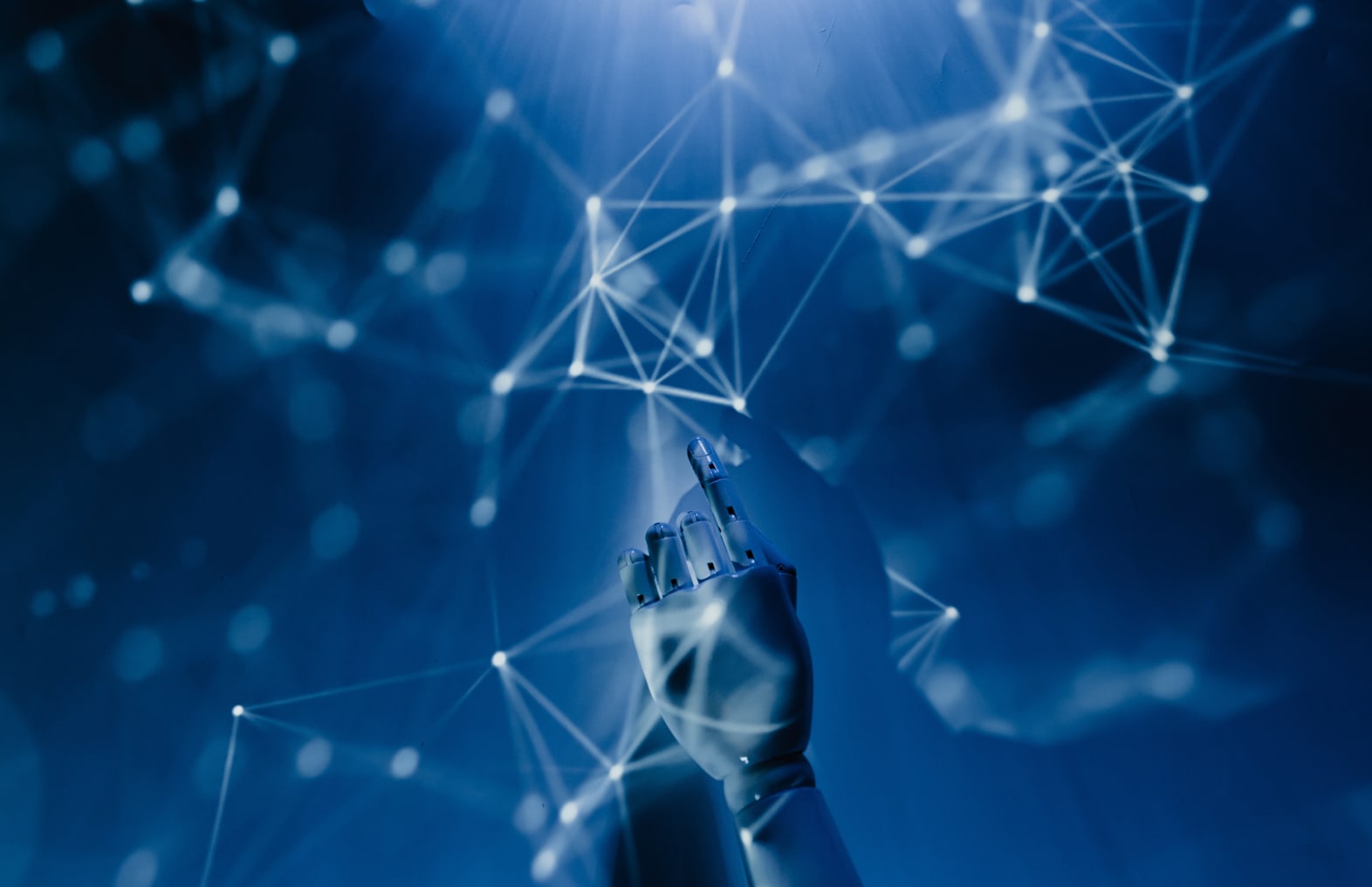
Today we needed to check which VM’s in System Center Virtual Machine Manager (SCVMM) were having ISO’s mounted. In order to prevent us from having to check each of our 200+ virtual machines individually we decided to script this with powershell. This is the script we came up with:
param([string] $VMMServer = $(throw “Please specify a VMM-Server to connect to.”))
Get-VirtualDVDDrive -VMMServer $VMMServer -All | Where-object {$_.ISO -ne $null} | select-object Name, ISO, ISOLinked | Sort-object -Property Name
The script accepts one parameter, the VMM server, and returns the VM-Name, ISO-Name and ISOLinked, which tells you if the ISO is shared instead of copied.
Furthermore we wanted to dismount the ISO’s for all VM’s which had the vmguest-image mounted. Surprisingly enough when searching for a way to mount or unmount an ISO in SCVMM with powershell we didn’t get any results in google (but then again, maybe we didn’t look good enough).
Here’s a script to mount and dismount images to/from VM’s
# UnMount
param([string] $VMServer = $(throw “Please specify a VM to dismount media from.”))
get-virtualDVDDrive -VM $VMServer | set-VirtualDVDDrive -NoMedia
#Mount
param([string] $VMServer = $(throw “Please specify a VM to mount media to.”))
$ISO = get-ISO | where-object {($_.Name -eq ‘[YourISOFile].ISO’) -and ($_.LibraryGroup -eq ‘[YourLibrarygroup]’)}
get-virtualDVDDrive -VM $VMServer | set-VirtualDVDDrive -ISO $ISO -Link
In this script we used the librarygroup because we maintain copies of the same ISO’s in two library groups devided over two locations.
If you would then want to unmount the mounted vmguest images from all VM’s you would get something like this:
Get-VirtualDVDDrive -VMMServer $VMMServer -All | Where-object {($_.ISO -ne $null) -and ($_.ISOLinked -eq $false) -and ($_.ISO -like ‘vmguest’)} | Set-VirtualDVDDrive -NoMedia
Because of the ‘-and ($_.ISOLinked -eq $false)’ only those vmguest images which were not shared will be unmounted in this script.