Silverlight 4 vs Flex 4: Accessing the Clipboard
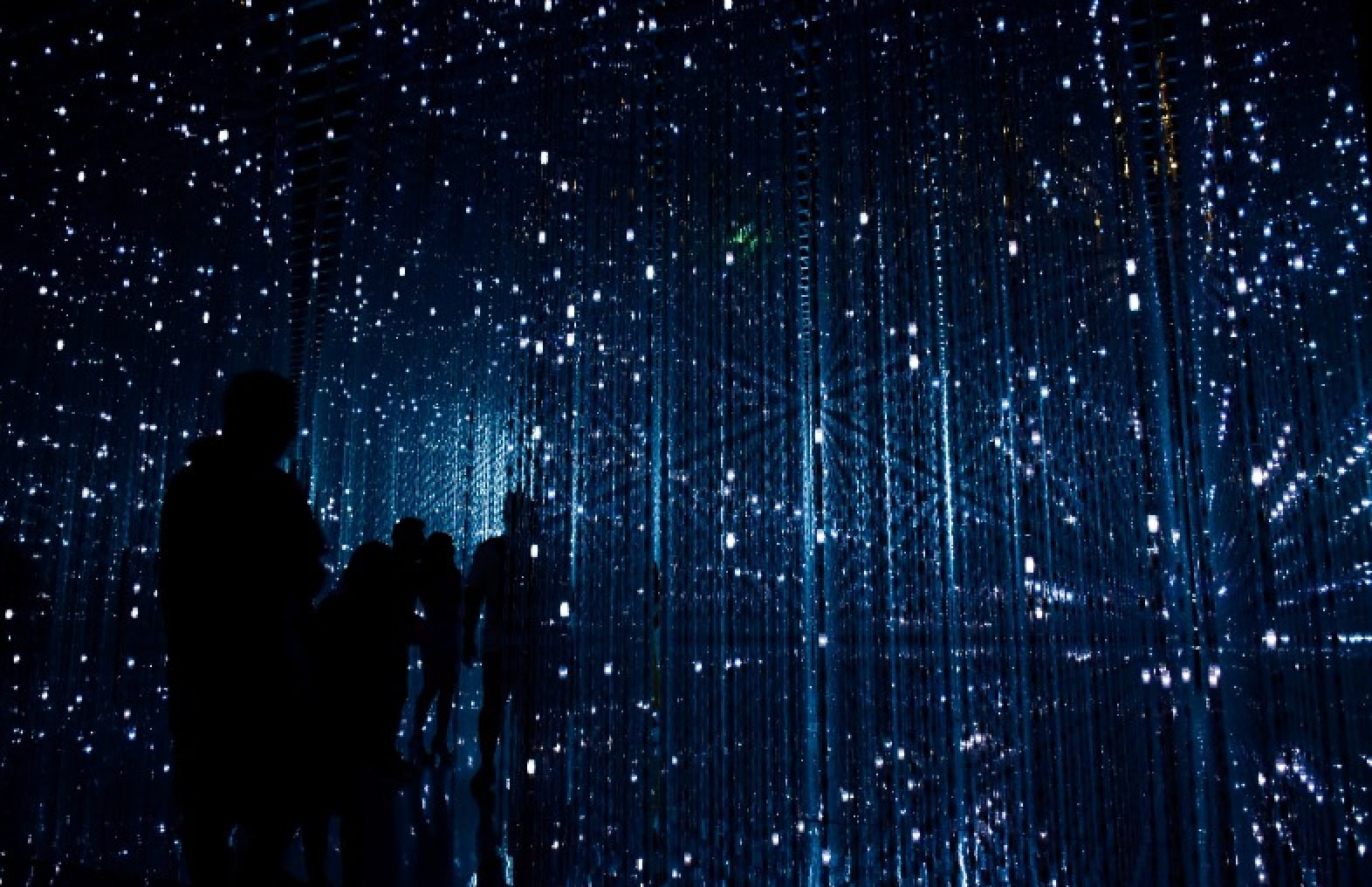
In this post I’m comparing Flex 4 and Silverlight 4’s clipboard access abilities. My previous post was rather long, so I’ll try to keep this one short :). Accessing the clipboard should be an interesting comparison, since both Flash 10 and Silverlight 4 take different approaches. I deliberately said “Flash 10” in the previous sentence, because accessing the clipboard in a Flex application is done by using the native Flash 10 ActionScript api and there isn’t any specific Flex code required.
Accessing the Clipboard in Flash 10
Accessing the clipboard in Flash has changed going from Flash player 9 to Flash Player 10. in Flash 9, one could set text on the user’s clipboard with the System.setClipboard() method from anywhere in the application. With Flash 10, this can only be done from a user initiated action. Also, while you can still use the System.setClipboard() method, you have more possibilities when using the new Clipboard class. Take a look at the user interface of the following Flex application:
The idea is simple: a user can enter text in the first textbox, press copy, then press paste to see the copied text in the second textbox. Sounds simple right? Yet this simple scenario could not be implemented with Flash. Let’s take look at the code:
1: <?xml version="1.0" encoding="utf-8"?>
2: <s:Application >"http://ns.adobe.com/mxml/2009"
3: >"library://ns.adobe.com/flex/spark"
4: >"library://ns.adobe.com/flex/halo" minWidth="1024" minHeight="768" creationComplete="setContextMenuPasteOnly(event)"
5: paste="handleApplicationPaste(event)">
6:
7: <fx:Script>
8: <![CDATA[
9: import mx.events.FlexEvent;
10: import flash.desktop.ClipboardFormats;
11: import flash.desktop.Clipboard;
12: protected function handleCopy(event:MouseEvent):void
13: {
14: Clipboard.generalClipboard.setData(ClipboardFormats.TEXT_FORMAT, _txtCopy.text);
15: }
16:
17: protected function handlePaste(event:MouseEvent):void
18: {
19: //throws error!
20: _txtPaste.text = String(Clipboard.generalClipboard.getData(ClipboardFormats.TEXT_FORMAT));
21: }
22:
23:
24: protected function setContextMenuPasteOnly(event:FlexEvent):void
25: {
26: var menu : ContextMenu = new ContextMenu();
27: menu.hideBuiltInItems();
28: menu.clipboardItems.paste = true;
29: menu.clipboardItems.selectAll = false;
30: menu.clipboardMenu = true;
31: contextMenu = menu;
32: }
33:
34:
35: protected function handleApplicationPaste(event:Event):void
36: {
37: _txtPaste.text = String(Clipboard.generalClipboard.getData(ClipboardFormats.TEXT_FORMAT));
38: }
39:
40: ]]>
41: </fx:Script>
42:
43:
44: <s:TextInput x="127" y="120" id="_txtCopy" />
45: <s:TextInput x="127" y="150" id="_txtPaste"/>
46: <s:Button x="263" y="120" label="Copy" click="handleCopy(event)"/>
47: <s:Button x="263" y="151" label="Paste" click="handlePaste(event)"/>
48:
49:
50: </s:Application>
The handleCopy() method on line 12 is the method that get’s called when a user clicks on the copy button.It copies the text in the first textfield using the static “generalClipboard” property of the Clipboard class. The setData() method accepts any object and you must specify the format for the object using the ClipboardFormats class. Quite a few formats are supported, but most of them are for AIR, Flash can only use TEXT_FORMAT,RICH_TEXT_FORMAT and HTML_FORMAT, of which the TEXT_FORMAT format is the most useful. The others are primarily for copy / pasting between Flash applications and they all boil down to text anyway.
The handlePaste() method on line 17 get’s called when a user clicks the paste button. Surprisingly enough, this method throws a SecurityError while I’m certainly in a user initiated action. It turns out that you can only read the clipboard content in a “paste” event, which is only fired when a user presses CTRL + V or clicks on the “paste” item in the the context menu that appears when a user right clicks a component in the Flash application.
Just to demonstrate how the paste event works, I’ve implemented it on the application level. Take a look on line 24 at the setContextMenuPasteOnly() method. This method is called in response to the “creationComplete” event of the application. It disables most of the items of the default Flash context menu and enables the so called “clipboardMenu”. Of the “clipBoardMenu” items, only the “paste” item is active. When the user right clicks the application (not the textbox) the following menu is shown:
When a user clicks “Paste” or presses CTRL + V, the application fires the “paste” event, when this happens the handleApplicationPaste() method on line 35 is called and the copied text is put in the second textbox. Note that most of the built in text components already have support for the “paste” item in the context menu and also support CTRL + V, all I have accomplished is that the user can press CTRL+V or right click anywhere in the application to paste the copied text. Pasting when the user clicks the button isn’t going to happen in Flash 10.
Accessing the Clipboard in Silverlight 4
Using the clipboard in Silverlight 4 is actually a lot easier than in Flash 10. Take a look at the following user interface:
Same idea as the Flex app, but in Silverlight I can implement this application as intended. Clipboard access in Silverlight is also done using the Clipboard class. This class has three important methods, which are actually self-explanatory:
- SetText()
- GetText()
- ContainsText()
This means that you can only set and read text from the clipboard in Silverlight, no other formats. The interesting part in Silverlight is, that you can actually access the clipboard from any user initiated event. When you access the clipboard the first time in a Silverlight application, the application asks for the user’s permission:
If not granted, the accessing of the clipboard throws a SecurityException. If granted, Silverlight won’t ask again until the next run of the application. Take a look at the code behind:
1: using System;
2: using System.Collections.Generic;
3: using System.Linq;
4: using System.Net;
5: using System.Windows;
6: using System.Windows.Controls;
7: using System.Windows.Documents;
8: using System.Windows.Input;
9: using System.Windows.Media;
10: using System.Windows.Media.Animation;
11: using System.Windows.Shapes;
12: using System.Security;
13:
14: namespace CopyPaste
15: {
16: public partial class MainPage : UserControl
17: {
18: public MainPage()
19: {
20: InitializeComponent();
21: }
22:
23: private void HandleCopy(object sender, RoutedEventArgs e)
24: {
25: try
26: {
27: Clipboard.SetText(_txtCopy.Text);
28: }
29: catch (SecurityException)
30: {
31: MessageBox.Show("You should allow clipboard access :)");
32: }
33: }
34:
35: private void HandlePaste(object sender, RoutedEventArgs e)
36: {
37:
38: if (Clipboard.ContainsText())
39: {
40: try
41: {
42: _txtPaste.Text = Clipboard.GetText();
43: }
44: catch (SecurityException)
45: {
46: MessageBox.Show("You should allow clipboard access :)");
47: }
48: }
49: }
50: }
51: }
As I said, accessing the clipboard in Silverlight is very simple.
Conclusion
Well, Silverlight is the winner for me. I do have to code more if I want to create a nice right mouse button menu like in Flash, or add CTRL + V support to the entire application, but the added flexibility that Silverlight offers by allowing me to use any user initiated event for accessing the clipboard, simply outweighs that. You can find the Flex 4 example here and the Silverlight 4 example here.