What’s new in Prism v4
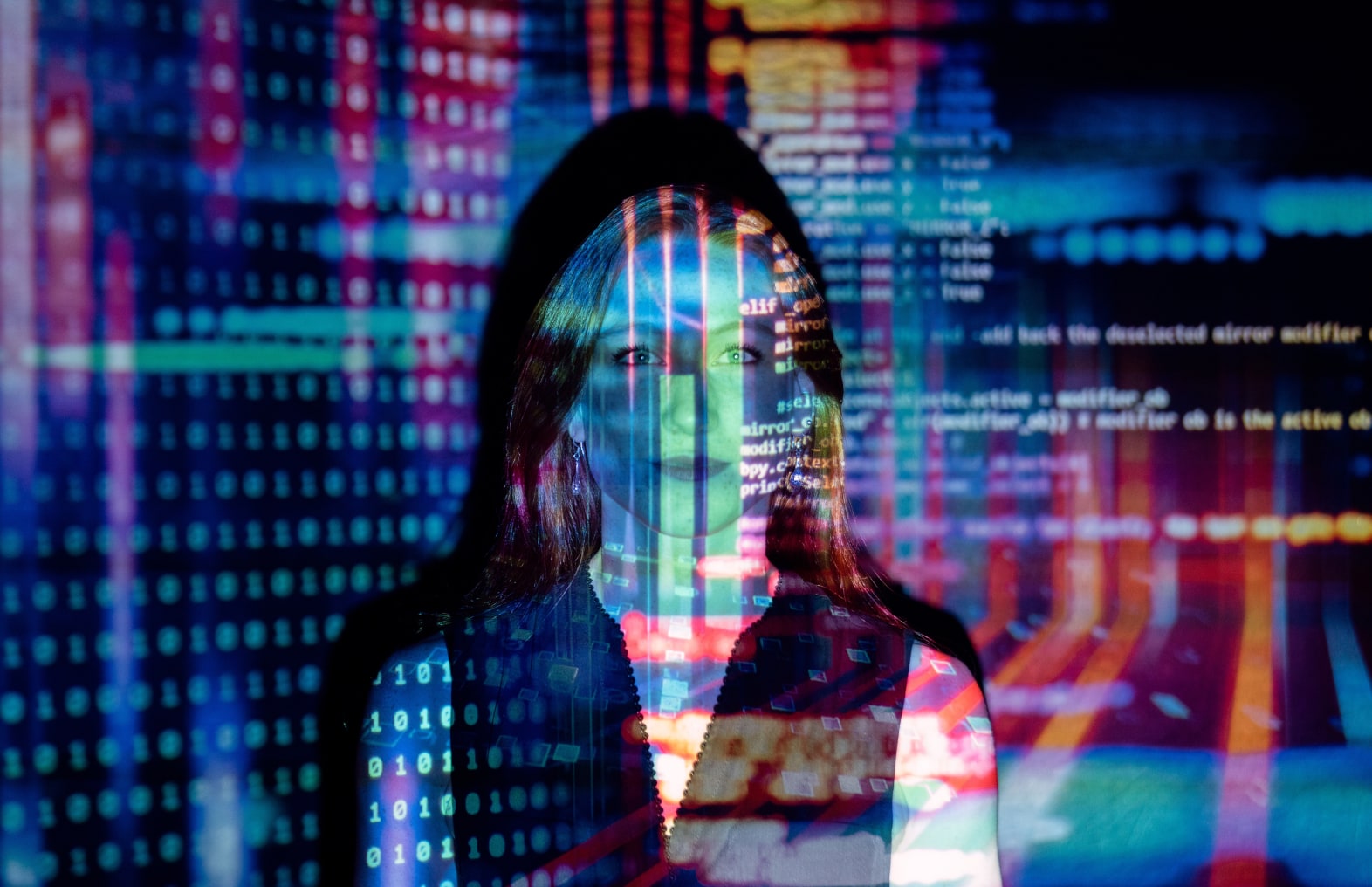
Building large Silverlight applications can be quite an undertaking. There’s loads of components to manage, so things can get out of hand when you don’t apply the right patterns to structure the application. Prism is a framework provided by Microsoft that helps you structure large Silverlight applications. It does so by doing two things, first it offers guidance on how to structure the components in the application and second it provides tools to make it easier to do so. In this article I will show you what’s in version 4 of the Prism framework.
MVVM guidance
The previous version of Prism featured guidance for the MVC and MVP design-patterns. The industry however has grown a strong liking for the MVVM pattern so Microsoft replaced the MVP and MVC guidance with a solid piece guidance on how to correctly apply MVVM with the various helper classes available in Prism.
The guidance describes the correct use of commands and how to correctly connect views to viewmodels. It also describes a very good method of interacting with the view from the viewmodel, without breaking the MVVM pattern called interaction requests.
Interaction requests
MVVM typically uses the notion of a view that knows the attached viewmodel. The viewmodel however does not need to know the view. This sometimes gives developers a headache,because sometimes you need access to the view from the viewmodel. Previously this wasn’t possible without some pretty hairy hacks. With the new version of Prism coming up Microsoft has created the InteractionRequest<T> class that makes it possible to interact with the UI from the viewmodel, without the need to know any of the view components.
The InteractionRequest works by making use of events. When you call the InteractionRequest from within the viewmodel the Raised event will be fired on the view. The InteractionRequest does require the use of actions and triggers to work correctly.
To use interaction requests you need the InteractionRequest<T> class and a couple of other things. First you need to create a new InteractionRequest property on the viewmodel which can be used to execute the interaction request.
public InteractionRequest<DeleteCustomerConfirmation> ConfirmationRequest { get; set; } public void DeleteCustomer(int customerID) { ConfirmationRequest.Raise(new DeleteCustomerConfirmation(customerID), DeleteCustomer); }
Next you need to add a trigger and action to the view attached to the viewmodel. The trigger will react to the Raised event of the InteractionRequest and execute the action you attached to the trigger.
<!-- XML namespace omitted --> <UserControl> <i:Interaction.Triggers> <prism:InteractionRequestTrigger SourceObject="{Binding ConfirmationRequest}"> <prism:PopupChildWindowAction> <DataTemplate> <Grid> <TextBlock Text="{Binding Content}"/> </Grid> </DataTemplate> </prism:PopupChildWindowAction> </prism:InteractionRequestTrigger> </i:Interaction.Triggers> </UserControl>
Sending data from the viewmodel to the new user interface is done through the use of an object that you can pass in as the context for the interaction request. You will get the object back after the interaction request is completed in the callback you provided when raising the interaction request. The complete code used in the view model is shown below.
public InteractionRequest<DeleteCustomerConfirmation> ConfirmationRequest { get; set; } private void DeleteCustomer(int customerID) { ConfirmationRequest.Raise(new DeleteCustomerConfirmation(customerID), DeleteCustomer); } private void DeleteCustomer(DeleteCustomerConfirmation confirmation) { if (confirmation.Confirmed) { //TODO: Delete customer } }
As you can see this is a very neat solution to show a confirmation dialog and react to the outcome of the dialog without breaking the MVVM pattern. You can even unit-test this interaction pattern by hooking up the Raised event of the interaction request inside your unit-test. From there you can execute the necessary logic to produce the desired test situation.
Managed extensibility framework
Prism v4 not only introduces guidance for using MVVM, it also introduces a new inversion of control mechanism. Unity is still supported, but you can also use MEF as a means to hook components in the application together. MEF is native to Silverlight 4 and .NET framework 4 which makes it an obvious choice for Microsoft to include support for this framework in Prism.
MEF allows you to export contracts with implementations attached to them that can be later imported into other components. MEF uses a so called part catalogs to keep track of exported components. These catalogs can be combined with a composition container which is responsible for satisfying any imports with matching exports as it encounters them when constructing a new instance of a type requested by the user.
Not only did Microsoft add MEF as the new inversion of control container, but they also included a few very neat trick. They added the ExportView attribute to make it easier to compose user interface from parts coming from different application modules. By exporting a view using the ExportView technique you tell Prism that there’s a view available to the application and in what region to put that view. While doesn’t help you in all cases it should make it easier to compose a user interface out of several child views.
API changes
With the added support for MEF Microsoft also improved the API in several locations. Most notabily are the changes to the bootstrapper classes that you need to use for booting a prism based application. Both the MEF bootstrapper and the unity bootstrapper have more points at which you can extend the bootstrapper logic. For example creating and initializing the Shell is now split in two. There’s a CreateShell method that you can override and a method called InitializeShell that you can use to initiliaze the shell. The extra extension points should give you more control over the application.
Upgrading from a previous edition
Already using Prism and want to upgrade? Microsoft has provided some additional documentation in the Prism helpfile to help you get up and running fast. Not only is there information on how to upgrade from a previous version of Prism. There’s also information on how to move from the composite UI application block to Prism.
It’s also good to know that upgrading from Prism v2 requires some minor changes to your code. For example, they shortened the name of some namespaces and renamed a few methods. There are no major changes in the class structure, so upgrading should be a breeze.
Conclusion
Prism v4 is not done, but the latest drop (Prism v4 drop 10) looks promising. Despite the huge change in direction from MVP/MVC to MVVM there are no major changes in the API to enable this. Everything you created using the previous version of Prism can be upgraded to the latest bits with little effort.
For more information check out the prism website: http://compositewpf.codeplex.com/